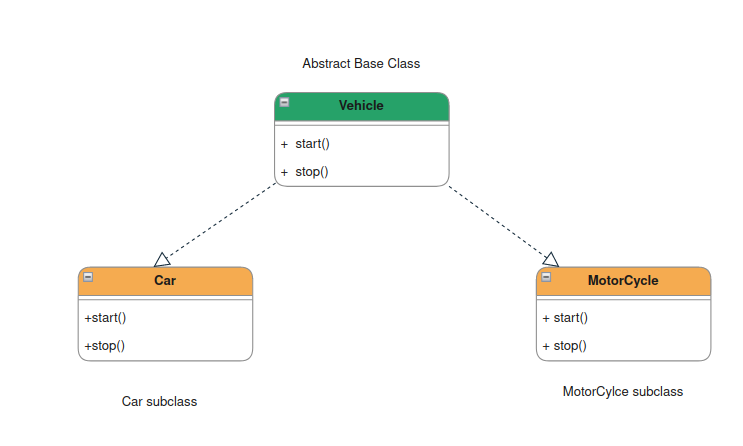
1. What Are Abstract Base Classes (ABCs)?
An Abstract Base Class (ABC) is a class that cannot be instantiated and is meant to be inherited by subclasses. It defines a blueprint for subclasses by enforcing the implementation of specific methods.
If a subclass does not implement the required methods, Python will throw an error. Think of ABCs as a contract—if a class inherits from an ABC, it must follow the rules.
Why is this useful?
- Ensures that all subclasses implement necessary methods.
- Prevents unexpected behavior when working with multiple developers.
- Improves code readability and maintainability.
If Python were a restaurant, ABCs would be the health inspectors ensuring all kitchens (subclasses) meet hygiene standards before serving food.
Error in Python 3.13:
SyntaxError: Missing ':' at the end of the function definition.
These enhanced messages save time and reduce frustration, especially for beginners.
2. Why Use ABCs?
Without ABCs, Python allows you to define a class with required methods, but it doesn’t enforce their implementation. This can lead to runtime errors when a method is called but hasn’t been implemented.
ABCs solve this problem by enforcing method implementation at the class definition stage, making errors easier to catch during development rather than at runtime.
Use cases where ABCs shine:
- Building frameworks: When creating a framework, you want to ensure that users of the framework follow required patterns.
- Plugin systems: If you are building a plugin system, you can enforce that all plugins implement specific methods.
- Consistent API design: When multiple developers work on the same project, ABCs help maintain uniformity across the codebase.
3. How to Implement ABCs in Python
Python provides the abc module to define abstract base classes. Here’s how you can create and use an ABC:
1. Define an Abstract Base Class
Use ABC from the abc module and the @abstractmethod decorator to define methods that must be implemented by subclasses.
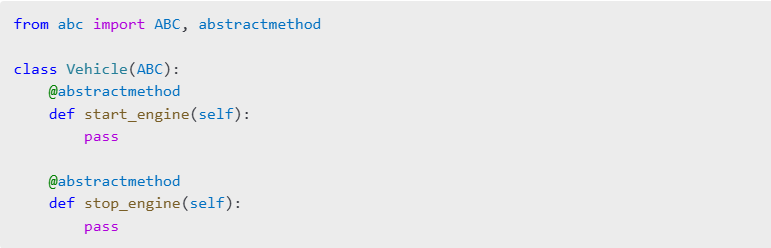
2. Implement the ABC in a Subclass
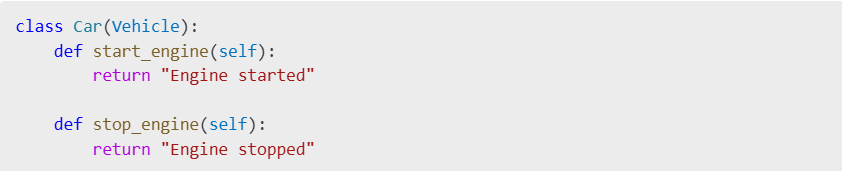
If a subclass does not implement all abstract methods, Python will raise an error when you try to instantiate it.
Now, you can create an instance of Car, but trying to instantiate Vehicle directly will result in an error.

If you’ve ever tried using an abstract class incorrectly, you know the frustration. It’s like ordering food at a self-service kiosk but realizing you never pressed “Confirm Order.” You’re just left waiting! 😉
4. Common Mistakes When Using ABCs
1. Forgetting to implement all abstract methods
- If a subclass doesn’t implement all abstract methods, it cannot be instantiated.
2. Trying to instantiate an ABC
- Abstract base classes cannot be instantiated directly. They serve as blueprints, not standalone objects.
3. Not using ABCs when needed
- If multiple classes share similar methods but don’t enforce their implementation, consider using ABCs to make your design more structured.
Conclusion
Abstract Base Classes (ABCs) in Python provide a structured approach to enforcing method implementation. They act as a contract, ensuring that subclasses implement necessary methods, improving code consistency, and preventing runtime errors.
If you’re working with frameworks, plugins, or large codebases, ABCs can make your code more maintainable and predictable. Just remember: ABCs are great for enforcing structure, but don’t overuse them where a simple base class would do the job.
In short, ABCs are like the traffic rules of Python. They may slow you down slightly, but they keep your project from crashing into chaos! 😉
Want to deepen your Python knowledge? Subscribe to our newsletter for expert insights and check out our Excel course!