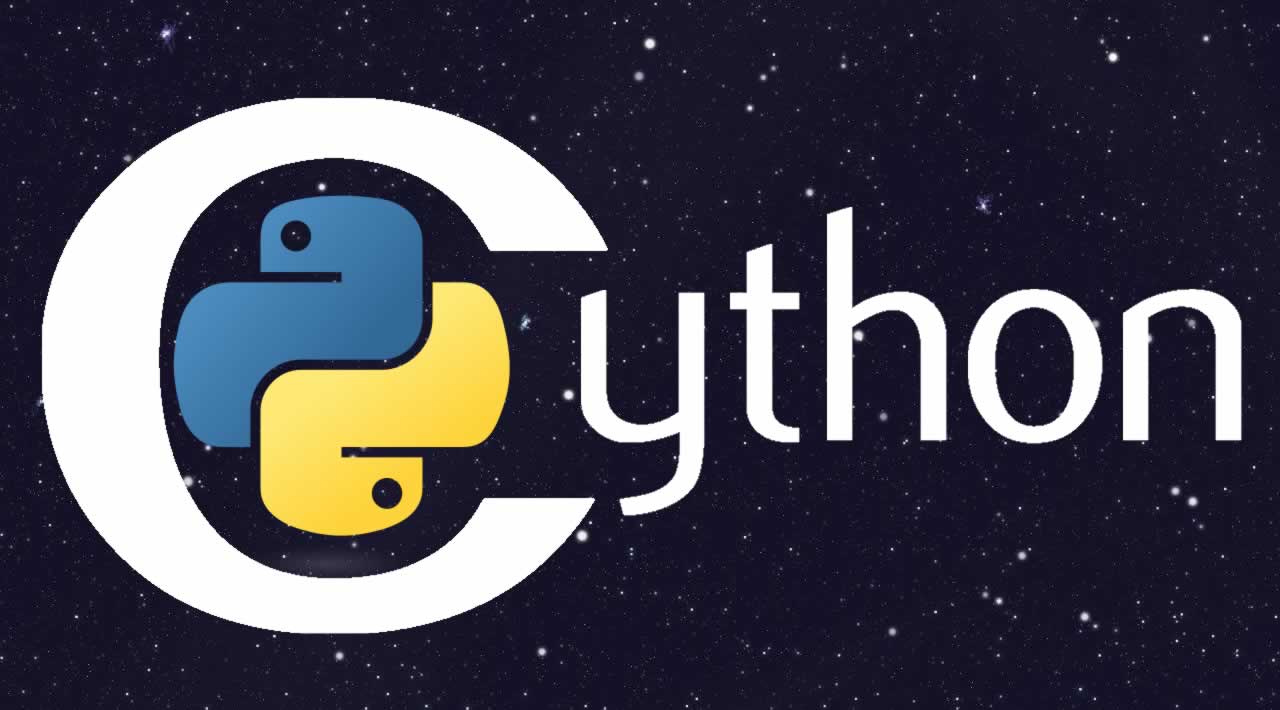
1. Improved Error Messages
In this blog, we’ll break down the major updates in Python 3.13, explore how they can benefit you, and provide examples to help you get started. By the end, you’ll understand why this release is being hailed as a game-changer.
Debugging is an inevitable part of coding. Python 3.13 introduces more user-friendly error messages, helping developers quickly identify and resolve issues.
- Better Syntax Error Details: When you encounter a syntax error, Python now provides clearer explanations, pinpointing the exact issue and suggesting potential fixes.
Example:
def func()
print("Hello, World!")
Error in Python 3.12:
SyntaxError: invalid syntax
Error in Python 3.13:
SyntaxError: Missing ':' at the end of the function definition.
These enhanced messages save time and reduce frustration, especially for beginners.
2. Faster Execution with CPython Optimization
Performance improvements are always welcome, and Python 3.13 delivers on this front with enhancements to CPython, the default Python interpreter.
- Key Improvements:
- Function calls are now up to 10% faster.
-Optimized memory usage reduces overhead for large datasets.
- Real-World Impact: Developers working on data-heavy applications or machine learning models will notice significant performance gains.
-Example: A script processing 1 million records runs noticeably faster in Python 3.13 compared to earlier versions.
3. New pattern Keyword for Pattern Matching
Pattern matching, introduced in Python 3.10, becomes more versatile with the new pattern keyword. This feature simplifies handling complex data structures like JSON objects or nested lists.
- Why It Matters: This update makes pattern matching more readable and expressive, reducing boilerplate code.
- Example:
data = {"status": "success", "code": 200}
match data:
case {"status": "success", "code": 200}:
print("Request was successful!")
case {"status": "error", "code": _}:
print("There was an error.")
This streamlined approach is ideal for handling APIs and configurations.
4. Enhanced Type Hints
Static typing in Python has become increasingly popular, and Python 3.13 introduces enhancements to type hints, making them more intuitive and expressive.
- What’s New:
- Support for generic types without importing from typing.
- Clearer syntax for union types using |.
- Example:
def add_numbers(a: int | float, b: int | float) -> float:
return a + b
print(add_numbers(5, 3.2)) # Works seamlessly
These improvements make your code easier to read and maintain, especially in large projects.
5. Expanded async Capabilities
Python 3.13 makes asynchronous programming more powerful by introducing new libraries and improving existing features.
- Notable Updates:
- asyncio.run() now supports timeouts directly.
- Improved task cancellation reduces the risk of resource leaks.
- Example:
import asyncio
async def fetch_data():
await asyncio.sleep(2)
return "Data received"
result = asyncio.run(asyncio.wait_for(fetch_data(), timeout=5)) print(result)
These updates make asynchronous programming smoother and more intuitive.
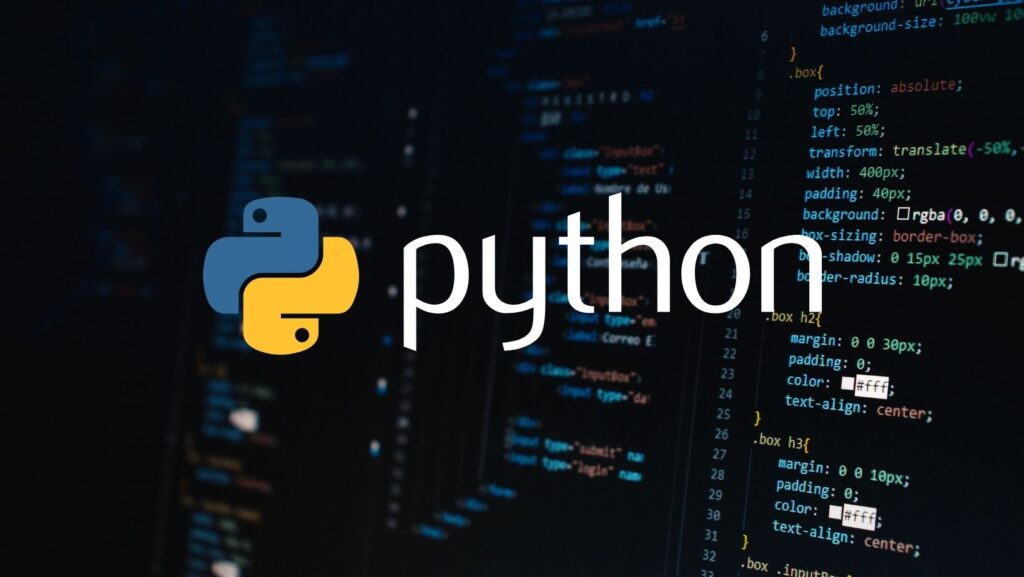
6. Smaller Quality-of-Life Updates
Python 3.13 also includes minor updates that enhance everyday coding experiences:
- Improved venv Module: Virtual environments now load faster and include default configurations for popular tools like lanterns.
- New Standard Libraries: Libraries like httpx and tomllib have been integrated, reducing dependency management.
- Example: With tomllib, you can now parse TOML files natively:
import tomllib
with open("config.toml", "rb") as f:
config = tomllib.load(f)
print(config["database"]["host"])
Final Thoughts: Why Upgrade to Python 3.13?
Python 3.13’s features address common pain points, from debugging to performance optimization, while introducing tools that make modern programming more accessible and efficient. Here’s why you should consider upgrading:
- Save time with clearer error messages.
- Boost application performance with CPython improvements.
- Write cleaner, more expressive code with enhanced pattern matching and type hints.
- Simplify asynchronous workflows with updated asyncio features.
If you found this blog useful, subscribe to our newsletter for more insights on Python, Excel, AI, and Power BI.